Introduction
FinovoTrader MT4/MT5 connector connects MetaTrader 4/5 with FinovoTrader. Using connector trades from MetaTrader can be sent to FinovoTrader. You automate an existing MetaTrader indicator to send orders whenever signal generated by indicator connector will detect it and send to FinovoTrader. You can also use connector API to send order from MetaTrader Expert to FinovoTrader. It's supported only for Binary Option brokers at the moment.
Installation
FinovoTrader MT4/MT5 connector installed by clicking Tools > Addons menu in FinovoTrader.
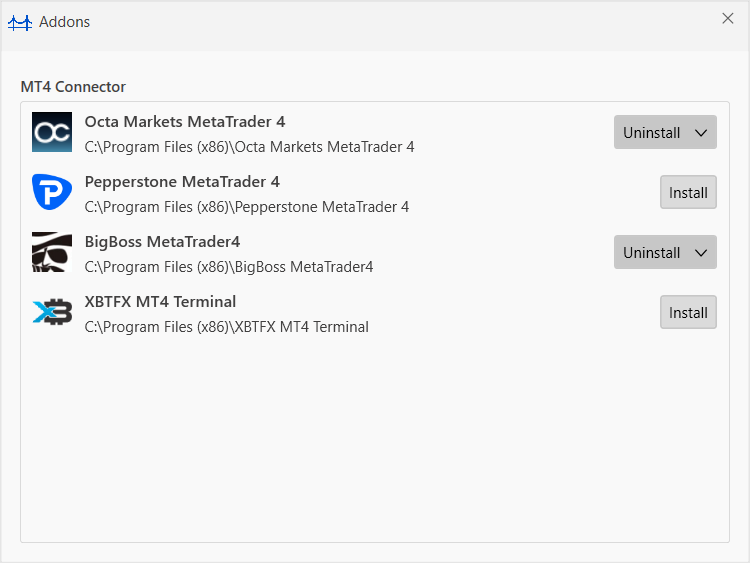
It will list all installed MetaTraders. Click Install button next to MetaTrader name for installation. Clicking Uninstall button will remove connector files from MetaTrader directory. Clicking Update will update connector files to latest version. Now connector is installed if MetaTrader is running restart otherwise open it.
Connector Options
Connector Options can be accessed by clicking Tools > Options > Connector.
Connector is disabled by default in FinovoTrader. To enable it check Connector Enabled.
Normal will send amount as defined.
Martingale will multiply amount by factor.
Trade Amount From Connector defines if amount will be used from MetaTrader order or this.
Fixed will use defined amount.
Percentage will use percent of account balance.
Send Order Automatically Checking it will send martingale order as soon as previous order closed in loss and max step not reached. Otherwise order will be use martingle when new order will be sent from MT Connector and previous order was in loss and max step not reached.
MaxStep defines maximum number of steps which need to send by martingale after reached amount will return to normal.
Factor factor defines value by which amount will be multiplied for martingale.
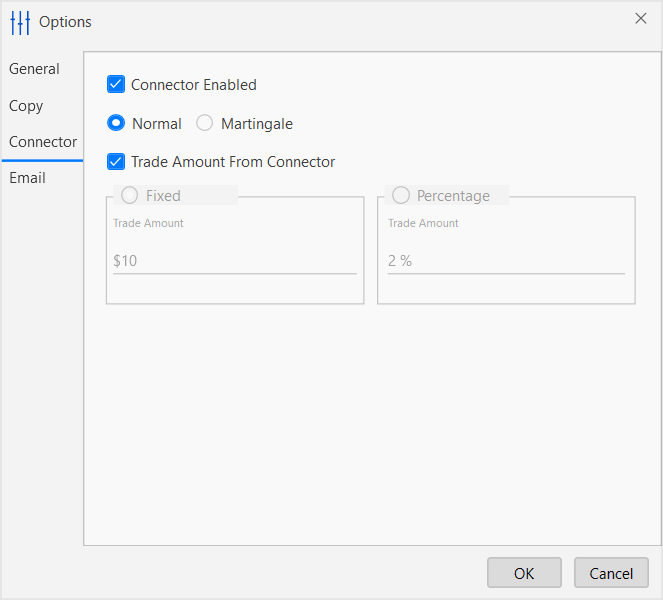
Indicator Auto Trading
Attach MetaTrader indicator to chart. Then from Expert Advisors list attach Finovo MT Auto Trader Expert to chart. Open Expert common tab and check Allow DLL imports to true. In Expert Inputs tab configure indicator for auto trading.
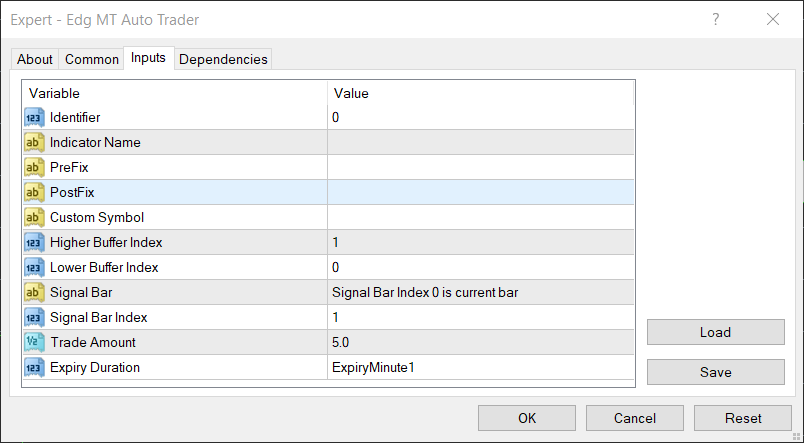
Inputs
Identifier can used to identify order uniquely.
Indicator Name is the name of indicator loaded to chart for trading.
PreFix is value before symbol if symbol name in MetaTrader not matches to broker symbol. You can enter this value so this value will removed from start of symbol.
PostFix is value after symbol if symbol name in MetaTrader not matches to broker symbol. You can enter this value so this value will removed from end of symbol.
Custom Symbol enter custom symbol name if you want to send order with this custom symbol name.
Higher Buffer Index is indicator buffer index for Higher/Call order. It's usually arrow below bar.
Lower Buffer Index is indicator buffer index for Lower/Put order. It's usually arrow above bar.
Signal Bar Index is indicator bar index. 1 means open order on next bar. 0 means on current bar open order as soon as signal received.
Trade Amount is amount of order.
Expiry Duration is option expiry time.
Now click OK button. If everything configured successfully you will all of these boxes green. If error occured then respective box will be red.
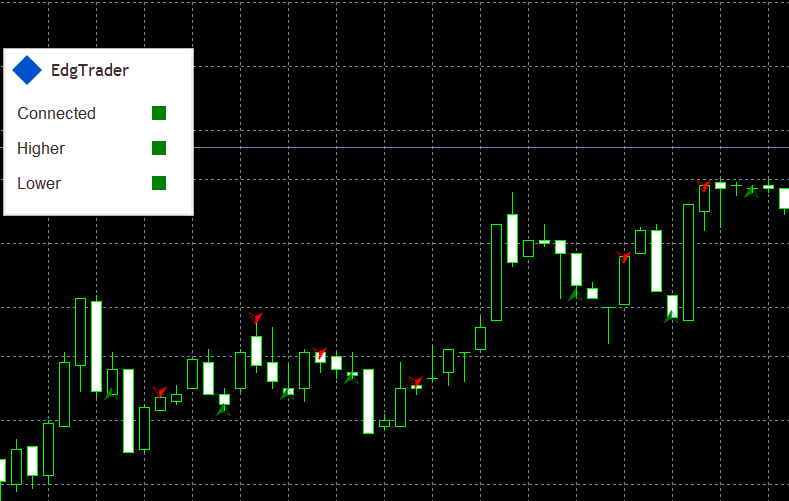
Connector API Trading
You can send order from Expert Advisor by using Connector API. All data types are defined in FinovoTraderLibrary.mqh.
Binary Broker Types
Order Types
enum BinaryOrderTypes
{
BinaryOrderRise,
BinaryOrderFall,
BinaryOrderRiseEqual,
BinaryOrderFallEqual,
BinaryOrderHigher,
BinaryOrderLower,
BinaryOrderTouch,
BinaryOrderDoesNotTouch,
BinaryOrderEndsBetween,
BinaryOrderEndsOutside,
BinaryOrderStaysBetween,
BinaryOrderGoesOutside
};
Duration Units
enum BinaryDurationUnits
{
BinaryDurationSeconds,
BinaryDurationMinutes,
BinaryDurationHours,
BinaryDurationDays,
BinaryDurationTicks
};
Base
enum BinaryBasis
{
BinaryBasePayout,
BinaryBaseStake
};
IQOption Data Types
Order Types
enum IqOrderTypes
{
IqOrderHigher,
IqOrderLower
};
Expiries
enum IqExpiresBinary
{
IqExpiryBinaryMinute1=1,
IqExpiryBinaryMinute2=2,
IqExpiryBinaryMinute3=3,
IqExpiryBinaryMinute4=4,
IqExpiryBinaryMinute5=5,
IqExpiryBinaryMinute15=6,
IqExpiryBinaryMinute30=7,
IqExpiryBinaryMinute45=8,
IqExpiryBinaryMinute60=9,
IqExpiryBinaryMinute75=10,
IqExpiryBinaryMinute90=11,
IqExpiryBinaryMinute105=12,
IqExpiryBinaryMinute120=13,
IqExpiryBinaryMinute135=14,
IqExpiryBinaryMinute150=15,
IqExpiryBinaryMinute165=16,
IqExpiryBinaryMinute180=17,
IqExpiryBinaryEOD=18,
IqExpiryBinaryEOW=19,
IqExpiryBinaryEOM=20
};
Common Data Types
Order Types
enum CommonOrderTypes
{
CommonOrderHigher,
CommonOrderLower
};
Expiries
enum CommonExpiries
{
CommonExpiryMinute1=1,
CommonExpiryMinute2 = 2,
CommonExpiryMinute3 =3,
CommonExpiryMinute4 =4,
CommonExpiryMinute5 = 5,
};
Function Definitions
bool BinarySendOrder(string Symbol, BinaryOrderTypes BinaryOrderType, BinaryBasis BinaryBase, double Amount, int Duration, BinaryDurationUnits BinaryDurationUnit, string Barrier = NULL, string Barrier2 = NULL, long Identifier = 0);
bool BinarySendOrder(string Symbol, BinaryOrderTypes BinaryOrderType, BinaryBasis BinaryBase, double Amount,long StartDateEpoch, int Duration, BinaryDurationUnits BinaryDurationUnit, string Barrier = NULL, string Barrier2 = NULL, long Identifier = 0);
bool BinarySendOrder(string Symbol, BinaryOrderTypes BinaryOrderType, BinaryBasis BinaryBase, double Amount, long EndDateEpoch, string Barrier = NULL, string Barrier2 = NULL, long Identifier = 0);
bool BinarySendOrder(string Symbol, BinaryOrderTypes BinaryOrderType, BinaryBasis BinaryBase, double Amount, long StartDateEpoch, long EndDateEpoch, string Barrier = NULL, string Barrier2 = NULL, long Identifier = 0);
bool IqSendOrderBinary(string Symbol, IqOrderTypes IqOrderType, double Amount,IqExpiresBinary Expiry, long Identifier = 0);
bool CommonSendOrder(string Symbol,CommonOrderTypes CommonOrderType, double Amount,CommonExpiries CommonExpiry, long Identifier = 0);
Binary Orders
Send Order
Binary Broker SendOrder with DurationUnit.
bool result1= BinarySendOrder("EURUSD" , BinaryOrderHigher, BinaryBaseStake, 1, 1, BinaryDurationMinutes, NULL, NULL, 0);
if(result1)
{
Print("Order opened successfully");
}
else
{
Print(GetLastErrorEdgTrader());
}
Send Order
Binary Broker SendOrder with StartDate and DurationUnit.
bool result1= BinarySendOrder("EURUSD", BinaryOrderHigher, BinaryBaseStake, 1, ((long)TimeCurrent())+500, 2, BinaryDurationMinutes, NULL, NULL, 0);
if(result1)
{
Print("Order opened successfully");
}
else
{
Print(GetLastErrorEdgTrader());
}
Send Order
Binary Broker SendOrder with ExpiryDate.
bool result1= BinarySendOrder("EURUSD", BinaryOrderHigher, BinaryBaseStake, 1, ((long)TimeCurrent())+500, NULL, NULL, 0);
if(result1)
{
Print("Order opened successfully");
}
else
{
Print(GetLastErrorEdgTrader());
}
Send Order
Binary Broker SendOrder with StartDate and ExpiryDate.
bool result1= BinarySendOrder("EURUSD", BinaryOrderHigher, BinaryBaseStake, 1, ((long)TimeCurrent())+400, ((long)TimeCurrent())+900, NULL, NULL, 0);
if(result1)
{
Print("Order opened successfully");
}
else
{
Print(GetLastErrorEdgTrader());
}
IQOption Orders
Send Order
IqOption Broker SendOrder Binary Type.
bool result= IqSendOrderBinary(Symbol(), IqOrderLower, 1, IqExpiryBinaryMinute1 ,0);
if(result)
{
Print("Order opened successfully");
}
else
{
Print(GetLastErrorEdgTrader());
}
Common Orders
Send Order
SendOrder order to any broker which is logged in.
bool result=CommonSendOrder("EURUSD", CommonOrderHigher, 1, CommonExpiryMinute1);
if(result)
{
Print("Order opened sucessfully");
}
else
{
Print(GetLastErrorEdgTrader());
}
Examples
Examples are available in Finovo MT Examples Script in MetaTrader. It's installed with MT Connector installation.